Heap is a special case of balanced binary tree data structure where the root-node key is compared with its children and arranged accordingly. If α has child node β then − key(α) ≥ key(β) As the value of parent is greater than that of child, this property generates Max Heap.
Table of Contents
What is heap algorithm?
Heap is a special case of balanced binary tree data structure where the root-node key is compared with its children and arranged accordingly. If α has child node β then − key(α) ≥ key(β) As the value of parent is greater than that of child, this property generates Max Heap.

What is heap implementation?
The heap is one maximally efficient implementation of an abstract data type called a priority queue, and in fact, priority queues are often referred to as “heaps”, regardless of how they may be implemented. In a heap, the highest (or lowest) priority element is always stored at the root.
How do you write heap algorithm?
To build a max heap, you: Assign it a value. Compare the value of the child node with the parent node. Swap nodes if the value of the parent is less than that of either child (to the left or right). Repeat until the largest element is at the root parent nodes (then you can say that the heap property holds).
What is heap explain with example?
A heap is a tree-based data structure in which all the nodes of the tree are in a specific order. For example, if is the parent node of , then the value of follows a specific order with respect to the value of and the same order will be followed across the tree.
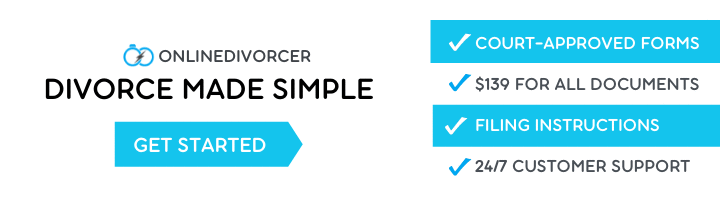
How heap sort is implemented?
Heap sort is a comparison-based sorting technique based on Binary Heap data structure. It is similar to selection sort where we first find the minimum element and place the minimum element at the beginning. We repeat the same process for the remaining elements.
How is heap implemented in data structure?
Heap Operations
- Let the input array be Initial Array.
- Create a complete binary tree from the array Complete binary tree.
- Start from the first index of non-leaf node whose index is given by n/2 – 1 .
- Set current element i as largest .
- The index of left child is given by 2i + 1 and the right child is given by 2i + 2 .
Which algorithm is used to implement heap sort?
Heapsort is not a stable algorithm. To implement heapsort, we make use of either min-heap or max-heap. We create a min-heap or a max-heap out of the given array elements and the root node is either the minimum or the maximum element respectively.
What is the purpose of heaps?
A heap is a binary tree data structure (see BinaryTrees) in which each element has a key (or sometimes priority) that is less than the keys of its children. Heaps are used to implement the priority queue abstract data type (see AbstractDataTypes), which we’ll talk about first.
Which algorithm is used in heap sort?
Heap sort is one of the sorting algorithms used to arrange a list of elements in order. Heapsort algorithm uses one of the tree concepts called Heap Tree. In this sorting algorithm, we use Max Heap to arrange list of elements in Descending order and Min Heap to arrange list elements in Ascending order.
What are heaps used for?
Which algorithm is used to implement heap sort Mcq?
Explanation: Heap sort is based on the algorithm of priority queue and it gives the best sorting time.
When should you use heaps?
Use it whenever you need quick access to the largest (or smallest) item, because that item will always be the first element in the array or at the root of the tree. However, the remainder of the array is kept partially unsorted. Thus, instant access is only possible to the largest (smallest) item.
How does heap sort algorithm work?
The heapsort algorithm involves preparing the list by first turning it into a max heap. The algorithm then repeatedly swaps the first value of the list with the last value, decreasing the range of values considered in the heap operation by one, and sifting the new first value into its position in the heap.
Where is heap used in real life?
Taking an example from real life —Heap Sorting can be applied to a sim card store where there are many customers in line. The customers who have to pay bills can be dealt with first because their work will take less time. This method will save time for many customers in line and avoid unnecessary waiting.
On which algorithm is heap sort based?
Why do we need a heap?
You should use heap when you require to allocate a large block of memory. For example, you want to create a large size array or big structure to keep that variable around a long time then you should allocate it on the heap.