Example to change TitleBar icon in Java Swing
Table of Contents
How do I change the default Java icon in JFrame?
Example to change TitleBar icon in Java Swing

- import javax.swing.*;
- import java.awt.*;
- class IconExample {
- IconExample(){
- JFrame f=new JFrame();
- Image icon = Toolkit.getDefaultToolkit().getImage(“D:\\icon.png”);
- f.setIconImage(icon);
- f.setLayout(null);
What are three JFrame parts?
We will write these classes independently here, but sometimes using inner classes simplifies their code. Basically, a JFrame represents a framed window and a JPanel represents some area in which controls (e.g., buttons, checkboxes, and textfields) and visuals (e.g., figures, pictures, and even text) can appear.
What are the parameters of the JFrame’s setSize method?
More JFrame size information
- setSize – Resizes this component so that it has width w and height h.
- setMinimumSize – Sets the minimum size of this window to a constant value.
- setPreferredSize – Sets the preferred size of this component to a constant value.
How do I change the look of Java Swing?
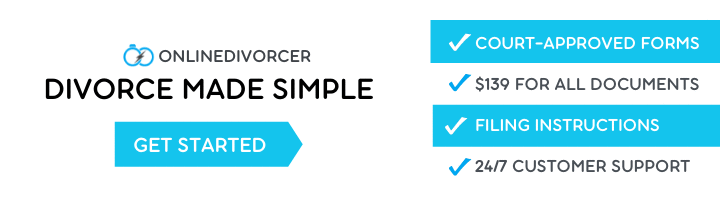
Swing Examples – Change the look and feel of a window
- UIManager. getCrossPlatformLookAndFeelClassName() − To get the Look and Feel of Java.
- UIManager. setLookAndFeel() − To set the look and feel of UI components.
- JFrame. setDefaultLookAndFeelDecorated(true); − To change the look and feel of the frame.
What are JFrame methods?
Method Summary
Modifier and Type | Method and Description |
---|---|
protected void | frameInit() Called by the constructors to init the JFrame properly. |
AccessibleContext | getAccessibleContext() Gets the AccessibleContext associated with this JFrame. |
Container | getContentPane() Returns the contentPane object for this frame. |
How do I customize a JFrame?
Creating a customized JFrame
- Create an undecorated JFrame with 60% opacity.
- Soft window edge. Referring to the image above, you can see that the edges are sharp and well-defined.
- Give it a rounded-rectangle shape. I can give a shape using AWTUtilities.
- Create a reflection of the `BufferedImage` to be used as background.
What is setDefaultCloseOperation JFrame Exit_on_close?
Calling setDefaultCloseOperation(EXIT_ON_CLOSE) does exactly this. It causes the application to exit when the application receives a close window event from the operating system.
What happens if setSize is not called on JFrame?
What happens if setSize is not called on a window? 1) The window is displayed at its preferred size. 2) It is a syntax error. 3) The window is not displayed.
Is JFrame Java Swing?
The class JFrame is an extended version of java. awt. Frame that adds support for the JFC/Swing component architecture.
What are different methods to create a frame?
Ways to create a frame:
- Methods:
- Way 1: By creating the object of Frame class (association)
- Example:
- Way 2: By extending Frame class (inheritance)
- Example:
- Output:
- Way 3: Create a frame using Swing inside main()
- Example 1:
Why do we use setDefaultCloseOperation in Java?
Calling setDefaultCloseOperation(EXIT_ON_CLOSE) causes the application to exit when the application receives a close window event from the operating system. Pressing the close (X) button on your window causes the operating system to generate a close window event and send it to your Java application.